Node、Java相互使用AES-256-CBC对数据进行加密解密实现
java中国官方JDK默认支持AES-128,在 1.8.0_151 和 1.8.0_152 版本之前,需要从Oracle官网下载安全JAR包方可支持AES-256,之后版本则可以通过在运行环境中设置以下属性启用AES-256支持!否则就会遇到这个错误:java.security.InvalidKeyException: Illegal key size。
1
| Security.setProperty("crypto.policy", "unlimited");
|
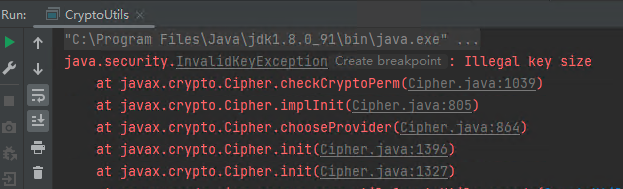
JDK7下载:Java Cryptography Extension (JCE) Unlimited Strength Jurisdiction Policy Files 7 Download
JDK8下载:JCE Unlimited Strength Jurisdiction Policy Files for JDK/JRE 8 Download
将压缩包中的文件替换${JAVA_HOME}/jre/lib/security
中的local_policy.jar
和US_export_policy.jar
即可
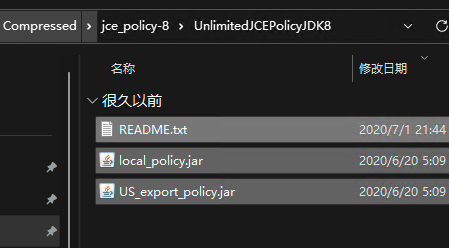
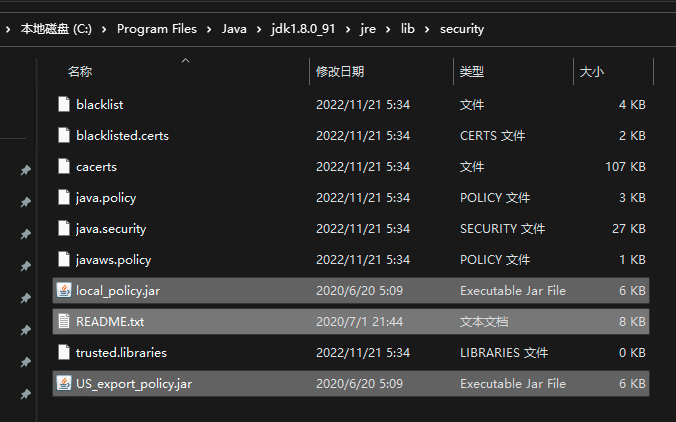
一、node
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47
| const crypto = require('crypto');
const algorithm = 'aes-256-cbc';
const key = '01234567890123456789012345678912';
const iv = '0123456789012345';
const charset = 'utf8';
const cipherEncoding = 'hex';
function encrypt(str) { let cipher = crypto.createCipheriv(algorithm, key, iv); let crypted = cipher.update(str, charset, cipherEncoding); crypted += cipher.final(cipherEncoding); crypted = crypted.replace(/\+/g, '%2b') return crypted }
function decrypt(str) { str = str.replace(/(%2b)/g, '+') let cipher = crypto.createDecipheriv(algorithm, key, iv); const decrypted = cipher.update(str, cipherEncoding, charset); result = decrypted + cipher.final(charset); return result }
function example(){ const str = 'hello world' const e = encrypt(str) console.log(`${str} 加密后:${e}`) const d = decrypt(e) console.log(`${str} 解密后:${d}`) }
example()
|
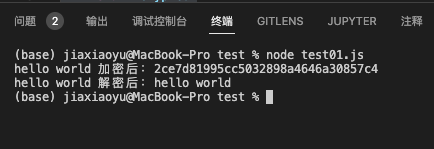
二、java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108
| package cn.yuencode.oisp.common.core.util;
import org.apache.commons.codec.binary.Hex;
import javax.crypto.Cipher; import javax.crypto.SecretKey; import javax.crypto.spec.IvParameterSpec; import javax.crypto.spec.SecretKeySpec; import java.util.Base64; import java.util.Base64.Decoder; import java.util.Base64.Encoder;
public class CryptoUtils {
private static final String key = "01234567890123456789012345678912";
private static final String iv = "0123456789012345";
private static final String cipher_algorithm = "AES/CBC/PKCS5Padding";
private static final String algorithm = "AES";
private static final String charset = "UTF-8";
private static final String cipherEncoding = "hex";
private static final Encoder base64Encoder = Base64.getEncoder(); private static final Decoder base64Decoder = Base64.getDecoder();
static { java.security.Security.setProperty("crypto.policy", "unlimited"); }
public static String encode(String content) { try { SecretKey secretKey = new SecretKeySpec(key.getBytes(), algorithm); Cipher cipher = Cipher.getInstance(cipher_algorithm); cipher.init(Cipher.ENCRYPT_MODE, secretKey, new IvParameterSpec(iv.getBytes()));
byte[] byteEncode = content.getBytes(charset);
byte[] byteAES = cipher.doFinal(byteEncode);
if ("hex".equalsIgnoreCase(cipherEncoding)) { return Hex.encodeHexString(byteAES); } else if ("base64".equals(cipherEncoding)) { return base64Encoder.encodeToString(byteAES); } } catch (Exception e) { e.printStackTrace(); } return null; }
public static String decode(String content) { try { SecretKey secretKey = new javax.crypto.spec.SecretKeySpec(key.getBytes(), algorithm); Cipher cipher = Cipher.getInstance(cipher_algorithm); cipher.init(Cipher.DECRYPT_MODE, secretKey, new IvParameterSpec(iv.getBytes())); byte[] byteContent = null; if ("hex".equalsIgnoreCase(cipherEncoding)) { byteContent = Hex.decodeHex(content); } else if ("base64".equals(cipherEncoding)) { byteContent = base64Decoder.decode(content); } byte[] byteDecode = cipher.doFinal(byteContent); return new String(byteDecode, charset); } catch (Exception e) { e.printStackTrace(); } return null; }
public static void main(String[] args) { String str = "hello world"; String str1 = encode(str); System.out.println(str + " 加密后:" + str1); String str2 = decode(str1); System.out.println(str + " 解密后:" + str2); } }
|
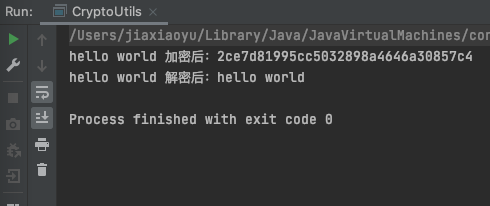